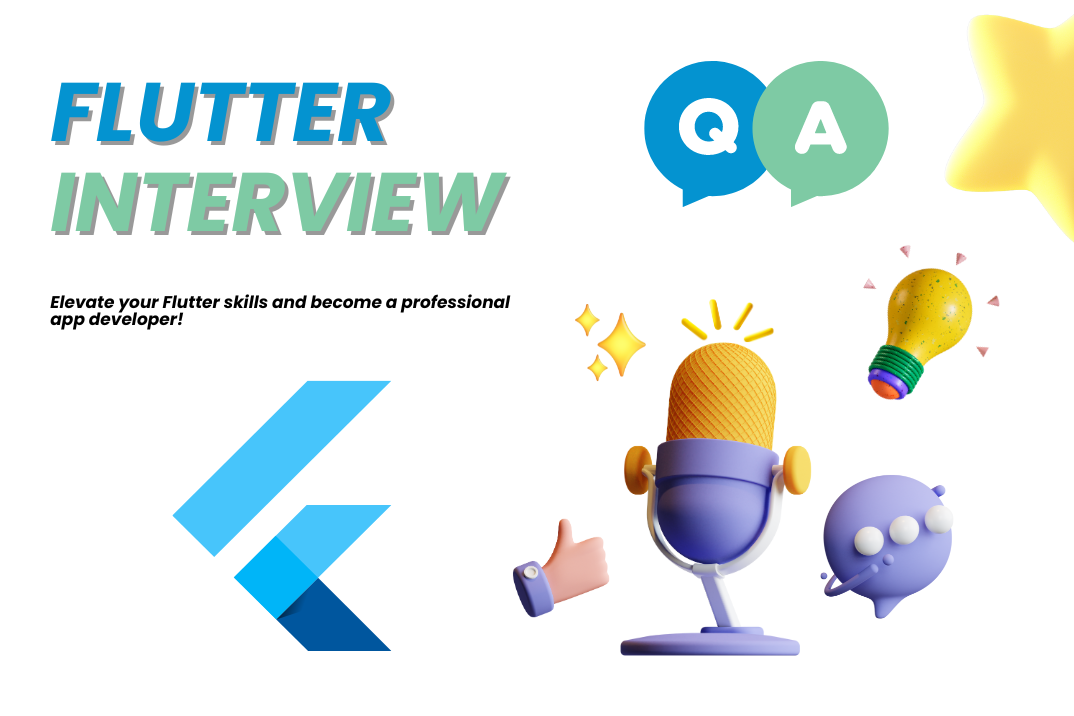
Flutter Interview Questions FAQ in 2023
- What is Flutter?
- • Flutter is an open-source UI framework developed by Google. It allows developers to build natively compiled applications for mobile, web, and desktop using a single codebase.
- What are the advantages of using Flutter for app development?
Some advantages of Flutter include:- • Fast development and hot reload feature
- • Single codebase for multiple platforms
- • Native performance and look
- • Rich set of customizable widgets
- • Access to native device features and APIs
- • Strong community support
- Explain the difference between Flutter and React Native.
Flutter and React Native are both popular frameworks for building cross-platform mobile apps. The main difference is that Flutter uses Dart as its programming language, while React Native uses JavaScript. Flutter compiles its code to native machine code, providing better performance, whereas React Native uses a bridge to communicate with native components.
- What is the programming language used in Flutter?
Flutter uses Dart as its primary programming language. Dart is an object-oriented language developed by Google, which is known for its simplicity, efficiency, and ease of learning.
- What is a widget in Flutter?
-
In Flutter, a widget is a UI component used to build the user interface of an application. Widgets can represent either a structural element (like a button or container) or a stylistic element (like padding or color). Widgets can be nested to create complex UI layouts.
-
- What is the difference between stateful and stateless widgets?
-
Stateless widgets are immutable and do not maintain any state. They are built only once and do not change during the lifetime of the application. Stateful widgets, on the other hand, can hold mutable state that can be updated and rebuilt over time. They are useful for handling user input and managing dynamic UI changes.
-
- What is the purpose of the setState() method?
-
The setState() method is used in Flutter to update the state of a stateful widget. When called, it notifies the framework that the internal state has changed, triggering a rebuild of the widget's UI. This allows developers to update the user interface dynamically in response to user actions or other events.
-
- What is a BuildContext in Flutter?
-
BuildContext is an object that holds contextual information about the widget tree. It is used to access various properties and functionalities of the surrounding widgets, such as finding the nearest ancestor of a specific type or accessing localized resources.
-
- . Explain the concept of hot reload in Flutter.
-
Hot reload is a feature in Flutter that allows developers to make changes to the source code and instantly see the results without restarting the entire application. It significantly speeds up the development process by providing a quick feedback loop.
-
- What is the purpose of the async and await keywords in Dart?
-
In Dart, the async keyword is used to mark a function as asynchronous, meaning it can perform tasks concurrently. The await keyword is used within an async function to pause the execution and wait for the completion of an asynchronous operation, such as an API call or file I/O.
-
- Explain the concept of Flutter widgets being reactive.
-
In Flutter, widgets are reactive, which means they automatically update in response to changes in their properties or the state of the application. When the properties or state of a widget change, Flutter efficiently rebuilds only the necessary parts of the UI to reflect those changes.
-
- What is the purpose of the GestureDetector widget?
-
The GestureDetector widget in Flutter is used to recognize various user gestures, such as taps, swipes, or long presses. It wraps its child widget and provides callbacks for handling different gestures.
-
- What is the purpose of the Navigator widget in Flutter?
-
The Navigator widget in Flutter manages a stack of routes and facilitates navigation between different screens or pages in an application. It provides methods for pushing, popping, and replacing routes.
-
- Explain the concept of layout widgets in Flutter.
-
Layout widgets in Flutter are used to control the positioning and sizing of other widgets within the UI. Examples of layout widgets include Container, Row, Column, Stack, and Expanded. They allow developers to create flexible and responsive UI layouts.
-
- What is the purpose of the ListView widget?
-
The ListView widget in Flutter is used to display a scrollable list of widgets. It can be used for displaying a large number of items efficiently by only rendering the visible portion of the list. There are different types of ListView widgets available, such as ListView.builder, ListView.separated, and ListView.custom, each with its own use cases.
-
- What are keys in Flutter?
-
Keys are identifiers used to associate widgets with their underlying elements in the widget tree. They help Flutter differentiate between widgets and track changes efficiently, especially when updating lists or managing state.
-
- Explain the concept of Future and Stream in Dart.
-
In Dart, a Future represents a potential value or error that will be available at some point in the future. It is commonly used for asynchronous operations that have a single result. A Stream, on the other hand, represents a sequence of asynchronous events over time. It is useful for handling continuous data flows, such as real-time updates or user input streams.
-
- What is the purpose of the InheritedWidget in Flutter?
-
The InheritedWidget is a special type of widget in Flutter that allows the propagation of data down the widget tree to its descendants efficiently. It is used to share data that needs to be accessible by multiple widgets without passing it explicitly through the widget hierarchy.
-
- How can you handle orientation changes in Flutter?
-
To handle orientation changes in Flutter, you can override the onOrientationChange method of the StatefulWidget and update the UI accordingly. Alternatively, you can use the OrientationBuilder widget to rebuild parts of the UI based on the new orientation.
-
- What is the purpose of the MediaQuery widget?
-
The MediaQuery widget in Flutter provides information about the current device's screen size, orientation, and other characteristics. It allows developers to build responsive UIs that adapt to different device configurations.
-
- Explain the concept of Flutter's widget lifecycle.
The widget lifecycle in Flutter consists of several stages:
- 1.Creation: The widget is instantiated.
- 2.Initialization: The widget's properties and dependencies are set.
- 3.State insertion: The widget's state is initialized.
- 4.Build: The widget's UI is constructed.
- 5.Deactivation: The widget is removed from the widget tree but can be reinserted.
- 6.Destruction: The widget is permanently removed from the widget tree.
- . What is the purpose of the IndexedStack widget?
-
The IndexedStack widget in Flutter is used to stack multiple widgets on top of each other and show only one widget at a time based on its index. It is often used in scenarios where you want to switch between different views without rebuilding the entire UI.
-
- What is the purpose of the SnackBar widget?
-
The SnackBar widget in Flutter is used to display temporary messages or notifications at the bottom of the screen. It is commonly used to show feedback to the user after performing an action or to display simple alerts.
-
- How can you handle user input in Flutter?
-
User input in Flutter can be handled using various gesture detectors, such as GestureDetector, InkWell, or IconButton, which provide callbacks for different types of gestures like taps, swipes, or long presses. Additionally, you can use form widgets like TextField or Checkbox to handle specific input types.
-
- What is Flutter's widget testing framework?
-
Flutter provides a testing framework called flutter_test for writing unit and widget tests. It allows developers to test the UI and behavior of their Flutter widgets and ensure the correctness of their application.
-
- What is the purpose of the Hero widget in Flutter?
-
The Hero widget in Flutter is used for creating hero animations. It allows a widget to smoothly transition between two screens by animating the shared element between them. This can provide a visually pleasing and seamless user experience.
-
- Explain the concept of dependency injection in Flutter.
-
Dependency injection is a software design pattern used in Flutter to provide the dependencies of a widget from an external source. It helps in decoupling the dependencies and allows for easier testing and maintenance. Flutter provides various dependency injection libraries like get_it, provider, or injector to implement this pattern.
-
- How can you handle data persistence in Flutter?
In Flutter, data persistence can be achieved through different mechanisms:
- 1. Shared preferences: Used for storing key-value pairs.
- 2. SQLite database: Provides a local relational database.
- 3. File I/O: Used for reading and writing data to files.
- 4. Network requests: Interacting with remote APIs or services.
- 5. State management libraries: Some state management libraries like Provider or Riverpod offer built-in support for managing and persisting application state.
- What is the purpose of the PageRoute class in Flutter?
-
The PageRoute class in Flutter represents a route that transitions between two screens or pages. It provides methods and properties for controlling the animation, transitions, and behavior of the route.
-
- What is the purpose of the ClipRRect widget?
-
The ClipRRect widget in Flutter is used to clip its child widget with rounded corners. It is often used to create rounded borders or to clip images with rounded edges.
-
- What is the purpose of the BackdropFilter widget?
-
The BackdropFilter widget in Flutter applies a filter effect to its child widget. It is commonly used to blur or apply other visual effects to the content behind the widget, such as creating frosted glass effects.
-
- Explain the concept of Flutter plugins.
-
Flutter plugins are packages that provide access to native device features and APIs not available directly through Flutter. They allow developers to integrate platform-specific functionality into their Flutter applications, such as accessing the camera, using sensors, or interacting with device hardware.
-
- How can you handle app localization in Flutter?
-
Flutter provides internationalization (i18n) and localization (l10n) support through the intl package. Developers can define message catalogs for different languages, extract and generate localized strings, and dynamically switch between different locales at runtime.
-
- What is the purpose of the Transform widget?
-
The Transform widget in Flutter is used to apply transformations to its child widget, such as scaling, rotating, translating, or skewing. It allows developers to manipulate the position, size, and orientation of the child widget.
-
- What is the purpose of the CustomPaint widget?
-
The CustomPaint widget in Flutter provides a canvas on which you can draw custom shapes, lines, or other graphical elements. It is commonly used for creating custom charts, graphs, or complex illustrations.
-
- Explain the concept of Flutter's reactive state management.
-
Reactive state management in Flutter refers to the pattern of managing and updating the application state in a reactive and declarative manner. It involves using state management techniques like setState, InheritedWidget, or external state management libraries like Provider, Riverpod, GetX, or Bloc to handle state changes and propagate them efficiently throughout the widget tree.
-
- What is the purpose of the ValueListenableBuilder widget?
-
The ValueListenableBuilder widget in Flutter is used to listen to changes in a ValueListenable and rebuild its child widget in response to those changes. It is often used when you need to rebuild a specific part of the UI based on a single value.
-
- How can you handle animations in Flutter?
-
Animations in Flutter can be handled using the built-in animation framework, which provides classes like Animation, Tween, AnimatedWidget, and AnimationController. Additionally, Flutter offers animation libraries like flutter_animation_progressions, flare_flutter, or Rive for more advanced animations.
-
- Explain the concept of Flutter's FutureBuilder widget
-
The FutureBuilder widget in Flutter is used to handle asynchronous operations represented by a Future. It provides a way to build different UI states based on the state of the future (e.g., loading, success, error), allowing developers to handle asynchronous data fetching and rendering.
-
- What is the purpose of the Spacer widget?
-
The Spacer widget in Flutter is used to create flexible empty space within a Row or Column layout. It expands to fill the available space and pushes other widgets apart, helping in creating responsive and adaptive UIs.
-
- What is the purpose of the SingleChildScrollView widget?
-
The SingleChildScrollView widget in Flutter is used to provide a scrollable view for its single child widget. It is typically used when the content exceeds the available screen space, allowing users to scroll and view the entire content.
-
- What is the purpose of the NotificationListener widget?
-
The NotificationListener widget in Flutter allows developers to listen for and handle different notifications emitted by the widgets in the widget tree. It provides callbacks for intercepting and responding to specific notifications, such as scrolling events, focus changes, or text input events.
-
- Explain the concept of Flutter's InkWell widget.
-
The InkWell widget in Flutter is used to create interactive ink splashes or ripples in response to user gestures, such as taps or presses. It provides an easy way to add visual feedback to touch events.
-
- What is the purpose of the AnimatedContainer widget?
-
The AnimatedContainer widget in Flutter is used to animate changes in its properties, such as size, position, or color. It automatically transitions between the old and new values, providing a smooth animation effect.
-
- What is the purpose of the Cupertino widgets in Flutter?
-
The Cupertino widgets in Flutter provide a set of iOS-style widgets and visual designs that closely resemble the native iOS UI components. They are used for creating applications with an iOS look and feel.
-
- Explain the concept of Flutter's Sliver widgets.
-
Sliver widgets in Flutter are used for creating scrollable areas with variable-sized children. They are part of the CustomScrollView widget and allow developers to create complex scrolling effects, such as parallax headers, sticky headers, or lazy loading lists.
-
- What is the purpose of the ClipPath widget?
-
The ClipPath widget in Flutter is used to clip its child widget using a custom clipper. It allows developers to create complex clipping shapes, such as circles, polygons, or custom paths
-
- How can you handle responsive UI design in Flutter?
-
To handle responsive UI design in Flutter, you can use flexible layout widgets like Row, Column, Wrap, or Expanded to adapt to different screen sizes and orientations. You can also utilize media queries with the MediaQuery widget to adjust the UI based on the available screen space.
-
- What is the purpose of the PageRouteBuilder in Flutter?
-
The PageRouteBuilder in Flutter is used to create custom page route transitions and animations. It provides more control over the animation behavior and allows developers to define custom transitions between screens.
-
- How can you handle deep linking in Flutter?
-
In Flutter, you can handle deep linking by using the flutter_deep_linking package or similar packages. These packages allow you to define URL patterns and associated actions within your app, enabling navigation to specific screens based on the deep link URL.
-
- What is the purpose of the LayoutBuilder widget?
-
The LayoutBuilder widget in Flutter is used to obtain information about the parent widget's constraints during the build process. It allows developers to create dynamic layouts based on the available space.
-
- Explain the concept of Flutter's ValueNotifier and ChangeNotifier.
-
ValueNotifier and ChangeNotifier are classes in Flutter used for managing and notifying changes to a value or object. ValueNotifier is typically used for single values, while ChangeNotifier is used for managing more complex objects that need to notify listeners about changes.
-
- What is the purpose of the AnimatedIcon widget?
-
The AnimatedIcon widget in Flutter is used to animate icons based on predefined icon animations. It provides a convenient way to animate between different states or styles of icons.
-
- What is the purpose of the LayoutAnimationBuilder widget?
-
The LayoutAnimationBuilder widget in Flutter is used to animate the creation and removal of widgets in a layout. It provides a way to animate the addition or removal of widgets with customizable animations.
-
- Explain the concept of Flutter's ValueKey and GlobalKey.
-
ValueKey and GlobalKey are key classes in Flutter used to identify and differentiate between widgets in the widget tree. ValueKey is used for identifying widgets based on their values, while GlobalKey is used for referencing and interacting with widgets from anywhere in the widget tree.
-
- What is the purpose of the AnimatedSwitcher widget?
-
The AnimatedSwitcher widget in Flutter is used to animate the transition between two or more widgets when they are replaced in the widget tree. It provides a smooth animation effect during widget transitions.
-
- What is the purpose of the Spacer widget?
-
The Spacer widget in Flutter is used to create flexible empty space within a Row or Column layout. It expands to fill the available space and pushes other widgets apart, helping in creating responsive and adaptive UIs.
-
- How can you handle biometric authentication in Flutter?
-
To handle biometric authentication in Flutter, you can use packages like local_auth or flutter_biometric_authentication. These packages provide APIs to authenticate users using fingerprint, face, or other biometric data, allowing you to add an extra layer of security to your app.
-
- What is the purpose of the Dismissible widget?
-
The Dismissible widget in Flutter is used to implement swipe-to-dismiss functionality for a widget. It allows users to swipe horizontally to remove items from a list or dismiss a widget from the screen.
-
- Explain the concept of Flutter's accessibility features.
-
Flutter provides various accessibility features to ensure that apps are usable by people with disabilities. These features include semantic annotations, screen reader support, customizable text scaling, support for high contrast modes, and more. By following accessibility best practices, developers can create inclusive apps that are accessible to a wider range of users.
-
These are some of the top Flutter interview questions and answers in 2023. It's important to note that the field of Flutter development is constantly evolving, so it's essential to stay updated with the latest changes and advancements in the framework.